⛄ Snowberb ⛄ – 12-56 May 26
Im trying to do a multi-filter function on React, and I'm really stuck.
I have diverse filters:
and I also have a lot of objects with those properties and more, called
bonuses: IBonus[]
My objective is that I have to apply all these filters to the bonuses
object at once, depending on what the user selects, for example, bookies
could be bet365
, Sportium
etc, ganancia
could have a min
of 20€ and a max
of 100€ etc.
How can I do this?
I could only achieve 1 filter at a time, I have no idea how I can chain all of them25 Replies
You don't chain the filters
You could, but it's not necessary
Rather, compose the predicates
That is to say, you have a function which will take one of the filters and the object and returns true or false (this is the predicate)
Create one such a predicate for every filter
And then you can use all of these predicates in the
.filter
function, so only the objects for which true
will be returned for all of the predicates will be keptcould you explain this one more in depth please?
Can you send the interface
IBonus
This is the type of filter im talking about btw (styling not finished)
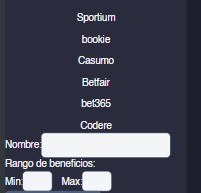
Imagine the state is
then you can create a predicate for each
then use these predicates as such
And you can AND (&&) all of these predicates for every filter you have in there
Be aware that if you have a filter that can be unselected / hold no value that the predicate should return true for those cases as well
This way
It would work only after hitting a button like "Apply filters"?
Would it be a lot more complex to filter
bonuses
every time a user selects a filter?That depends on when you choose to update the state, this part is filtering the state once the filters have been selected
mhm
Complex in terms of what?
difficulty
No it is not, it does not change the piece of code Im suggesting
That's about when you update the state
You can choose to update the state as the user clicks and updates each individual filter (controlled components) or you choose to only sync the state once the user clicks the button "apply filter"
waht im trying to say is that if you select for example
betfair365
then in bonuses
would only remain that house
and if I deselect that filter
i'd have no way of retrieving the other objectsDon't update the state of
bonuses
I make a copy of it?
You don't even have to
Just filter the
bonuses
state
bonuses
will always have all the bonusesoh I get it
There is no need to overwrite that state
you just lose information that way
true true
okay let me try this
thank you so much sebas
And what you're supposed to do is deriving the filtered view for your state
npnp
you have no idea how many times you helped me in this server 🤣
how do I print the array? If in my component
I'd have to make a copy of
bonuses
dont I? :/No,
.filter
will create a new array
Use that array
and last thing, what if I have the list paginated like this?
I should have said it before sorry, that's why I am asking if I should make a copy and how I must manage that
filteredItems
is a copy of bonuses
I have to go, hope someone else can help you from here
This thread hasn’t had any activity in 12 hours, so it’s now locked.
Threads are closed automatically after 12 hours. If you have a followup question, you may want to reply to this thread so other members know they're related. https://discord.com/channels/102860784329052160/565213527673929729/979367518831534140