Script – 22-55 Feb 1
I'm trying to run the function till
b.length === 1
and then return b
What am I doing wrong? it returns undefined
30 Replies
because you don't return
recall(b)
call?wow you could probably stand to name your variables a bit better 🙂
Like 

i meant this
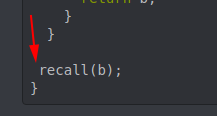
😂
I was just rushing and giving it similar var names
I tried that but still got undefined
is
b.length === 1
condition ever met?yes
so
recall
at that case should return non-undefined b
variable, because it surely is array/string with length property equal to 1I'll look at my code 

to be clear, you mean that
superDigit
returns undefined or recall
?
and are you sure that recursion ends sometime, not throws call stack exceeded error?Return the last line
return recall(b)
@ScriptOkay so I am finally exceeding the call stack
How do I manage this
Yeah then your base condition is never being met
It is actually
b becomes 3
that's length of 1
If you would properly hit the base condition you wouldn’t exceed the call stack
Are you calling length on a number? Does that give you the amount of digits?
yes b is an integer
What does the length of 0 return
0.length
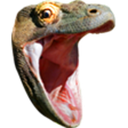
And
3.length
?
I’m on phone so I can’t verify myselfUnknown User•3y ago
Message Not Public
Sign In & Join Server To View
Are you perhaps assuming that you can call
length
on a number without actually trying it out?Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
Yeah
same thing
invalid
So why are you calling
length
on a number 😛
Turn it to a string first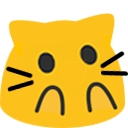
Don’t just assume code will work like you expect it to, you need to test and confirm even the smallest things heh
Yeah all good
ayee
still gettin undefined
wait am I supposed to return
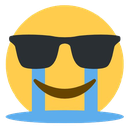
recall(b)
in recall()?
Because I did now and it workedYeah you are indeed
Since it will evaluate to a number, but that number needs to be returned to the caller
This question has an answer! Thank you for helping 😄
If you have a followup question, you may want to reply to this thread so other members know they're related. https://discord.com/channels/102860784329052160/565213527673929729/938205834796744794
This thread hasn’t had any activity in 12 hours, so it’s now locked.
Threads are closed automatically after 12 hours. If you have a followup question, you may want to reply to this thread so other members know they're related. https://discord.com/channels/102860784329052160/565213527673929729/938205834796744794