poppingpopper – 19-07 Oct 18
Hey! I'm trying to build a somewhat responsive page and I'm using a resize observer to get the width of the parent element to determine the width of my charts. For some reason the width changes in increments instead of immediately after the debounce interval I've set.
Can you help me figure out why this is happening and how I might fix it? (Also open to constructive criticism about the way I'm approaching it as well)
19 Replies
Here is the resize observer hook:
Here is the usage of the width returned
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
Yea, what do you want me to do exactly? Just print the width change out without the graphs present?
I think I'm experiencing a cascading effect where the width changes but the child content is choking it between width changes.
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
I'm trying to see if it's possible to get a deployed link but I don't konw yet.
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
The width is definitely being affected by the graphs as I'm only using the responsive width for the graphs. The width is being used like so in my charts
I'm listening to the parent container's dimensions
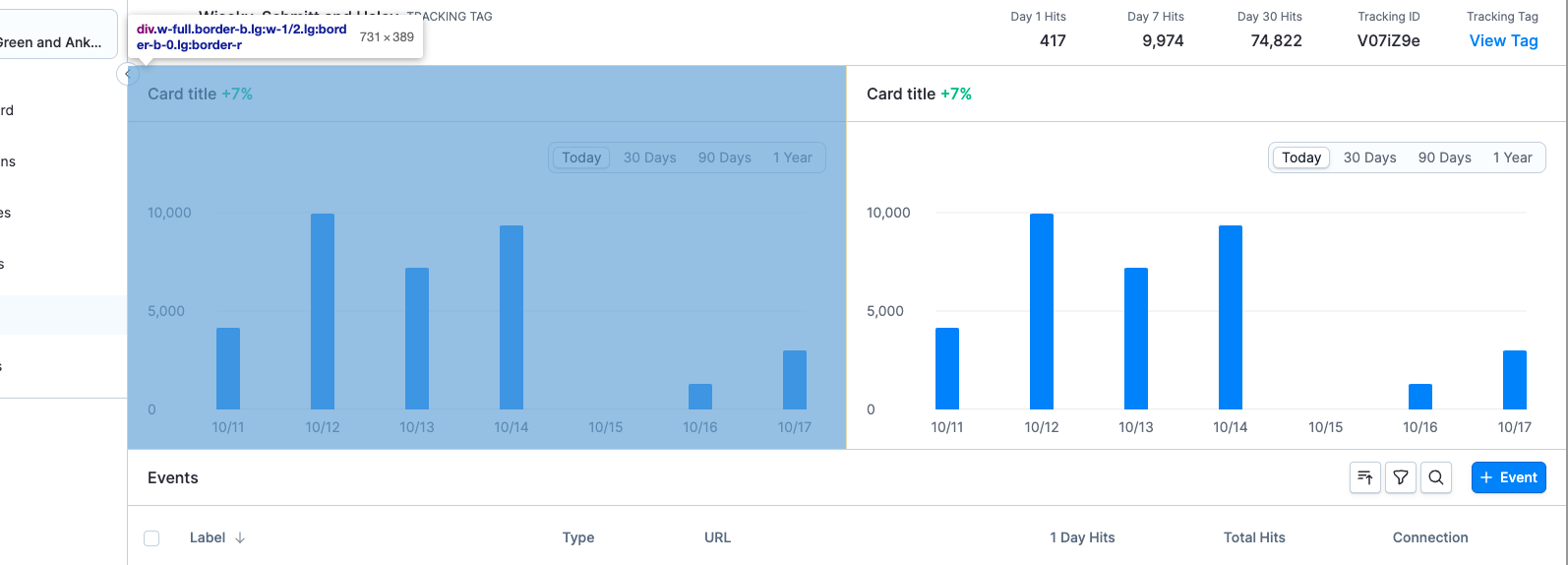
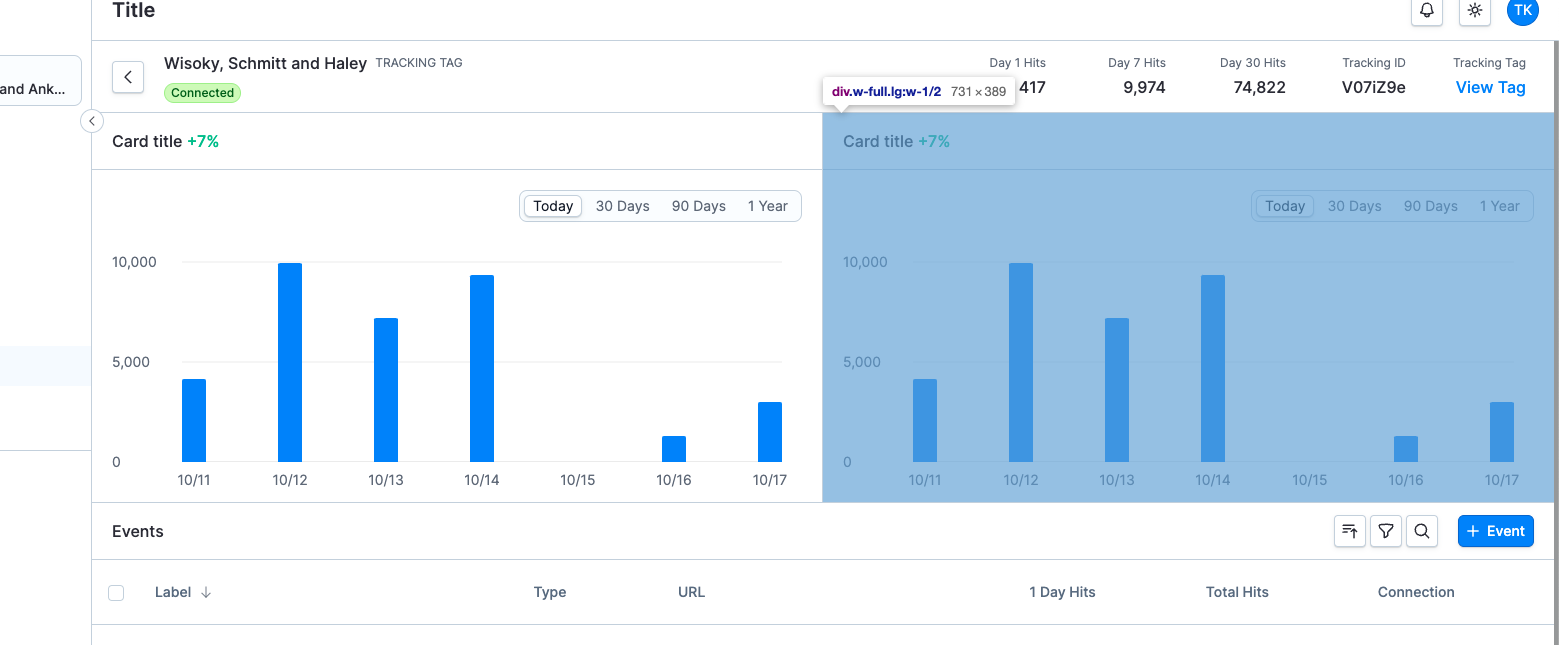
Solution
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
Haha! I am so dumb, I was thinking about what you said
i didnt get why this complexity instead giving w-full to parentThen I realized I used to have a reason for why I needed to listen to width changes. But not anymore. So I removed all that code and just let the width fill the flex container and its working. <:Laughing_Facepalm:1084929862810222622>
❤️ Thank you @oldcoder
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
@oldcoder How can I mark your message as the answer to this thread?
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
Thanks again!
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View
This thread hasn’t had any activity in 36 hours, so it’s now locked.
Threads are closed automatically after 36 hours. If you have a followup question, you may want to reply to this thread so other members know they're related. https://discord.com/channels/102860784329052160/902647189120118794/1164278522844549177