VuNguyen – 09-08 Apr 14
How do get process id using
ffi-napi
?
I'm using this code, which is generated by ChatGPT.
It works, but it just throw the error below randomly after getting some process id. I believe that this error is related to memory allocations.
5 Replies
These 3 lines of code caused the problem, but I have no idea how to fix it
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
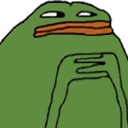
Unknown User•3y ago
Message Not Public
Sign In & Join Server To View
This thread hasn’t had any activity in 36 hours, so it’s now locked.
Threads are closed automatically after 36 hours. If you have a followup question, you may want to reply to this thread so other members know they're related. https://discord.com/channels/102860784329052160/565213527673929729/1096361353993793536